Teorema di Böhm-Jacopini
qualunque algoritmo può essere implementato in fase di programmazione (in diagramma di flusso, pseudocodice o codice sorgente) utilizzando tre sole strutture dette strutture di controllo: la sequenza, la selezione ed il ciclo (iterazione), da applicare ricorsivamente alla composizione di istruzioni elementari (ad esempio, istruzioni eseguibili con il modello di base della macchina di Turing).

Conditional Statement
Conditional statements are used to perform different actions based on different conditions. Very often when you write code, you want to perform different actions for different decisions. You can use conditional statements in your code to do this.
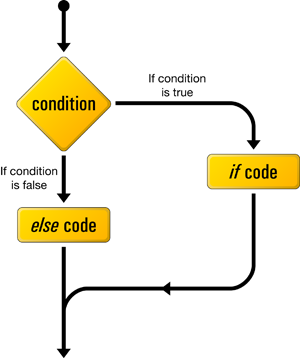
#!/bin/bash
if [ 1 == 1 ]
then
echo 1 is equal to 1
else
echo 1 is not equal to 1 #This part of code, will never be executed
fi
echo "program continue with other statements..."
if can be used in order to check if a file exists, or if a path exists.
#!/bin/bash
if [ -e ./testo1.txt ]
then
echo File testo1.txt exists
else
echo File testo1.txt does not exists
fi
echo "program continue with other statements..."
with unary operator, not
#!/bin/bash
if [ ! -e ./testoCheNonEsiste.txt ]
then
echo File testoCheNonEsiste does not exists
else
echo File testoCheNonEsiste.txt exists
fi
echo "program continue with other statements..."
With double condition or nested if
#!/bin/bash
if [ 1 == 1 ] && [ 2 == 2 ]
then
echo This file is useful.
else
echo "this part of code will never be true (in this case)"
fi
echo script go on...
Comparison between INTEGER and STRINGS, with elif ("else, if")
#!/bin/bash
# elif statements
if [ 2 -gt 1 ]
then
echo 2 is greater then 1
elif [ 'yes' == 'yes' ]
then
echo yes is always equal to yes, but we will never enter here
else
echo "This part of code, will never be executed (in this case)"
fi
Interger Comparison
-eq
is equal to
if [ "$a" -eq "$b" ]
-ne
is not equal to
if [ "$a" -ne "$b" ]
-gt
is greater than
#with double parentheses
if (("$a" > "$b"))
#with square parentheses
if [ "$a" -gt "$b" ]
-ge
is greater than or equal to
#with double parentheses
if (("$a" >= "$b"))
#with square parentheses
if [ "$a" -ge "$b" ]
-lt
is less than
#with double parentheses
if (("$a" < "$b"))
#with square parentheses
if [ "$a" -lt "$b" ]
-le
is less than or equal to
#with double parentheses
if (("$a" <= "$b"))
#with square parentheses
if [ "$a" -le "$b" ]
string comparison
= is equal to
if [ "$a" = "$b" ]
==
is equal to
if [ "$a" == "$b" ]
!=
is not equal to
if [ "$a" != "$b" ]
This operator uses pattern matching within a [[ ... ]] construct.
<
is less than, in ASCII alphabetical order
if [[ "$a" < "$b" ]]
if [ "$a" \< "$b" ]
Note that the "<" needs to be escaped within a [ ] construct.
>
is greater than, in ASCII alphabetical order
if [[ "$a" > "$b" ]]
if [ "$a" \> "$b" ]
Note that the ">" needs to be escaped within a [ ] construct.
-z
string is null, that is, has zero length
String='' # Zero-length ("null") string variable.
if [ -z "$String" ]
then
echo "\$String is null."
else
echo "\$String is NOT null."
fi # $String is null.
-n
string is not null.
Loops For
#!/bin/bash
for i in 1 2 3 4 5
do
echo "Loop number $i"
done
C Style
#!/bin/bash
for (( i=0; i<5; i++ ))
do
echo "Loop number $i"
done
While
#!/bin/bash
i=0
while [ $i -lt 5 ]
do
echo "Loop number $i"
i=$(( $i + 1 ))
done
Sum of 2 numbers, until variable "$a" will be -1
#!/bin/bash
while :
do
read -p "Enter two numnbers ( - 1 to quit ) : " a b
if [ $a -eq -1 ]
then
break #when condition is satisfied, the statement ends
fi
sum=$(( a + b ))
echo $sum
done
Let's iterate an array
#!/bin/bash
vet=("Stefano" "Marco" "Serena" "Mario")
# vet is and Array of 4 elements
for (( i=0; i<4; i++))
do
echo ${vet[i]}
if [ ${vet[i]} == 'Stefano' ]
then
echo "[ Comparazione stringa Avvenuta ]"
fi
done
#!/bin/bash
A=("9" "17" "stefano") #Array with no types declaration
#Array iteration with for loop
for i in ${A[@]}
do
echo ${i}
#And what would be happened if we'd do a math operation on array element, inside this loop?
done
What will be the value of "sum" at the end of the loop?
#!/bin/bash
A=("9" "17" "stefano") #no types declaration
for i in ${A[@]}
do
#echo ${i}
sum=$(($sum+${i}))
done
echo $sum